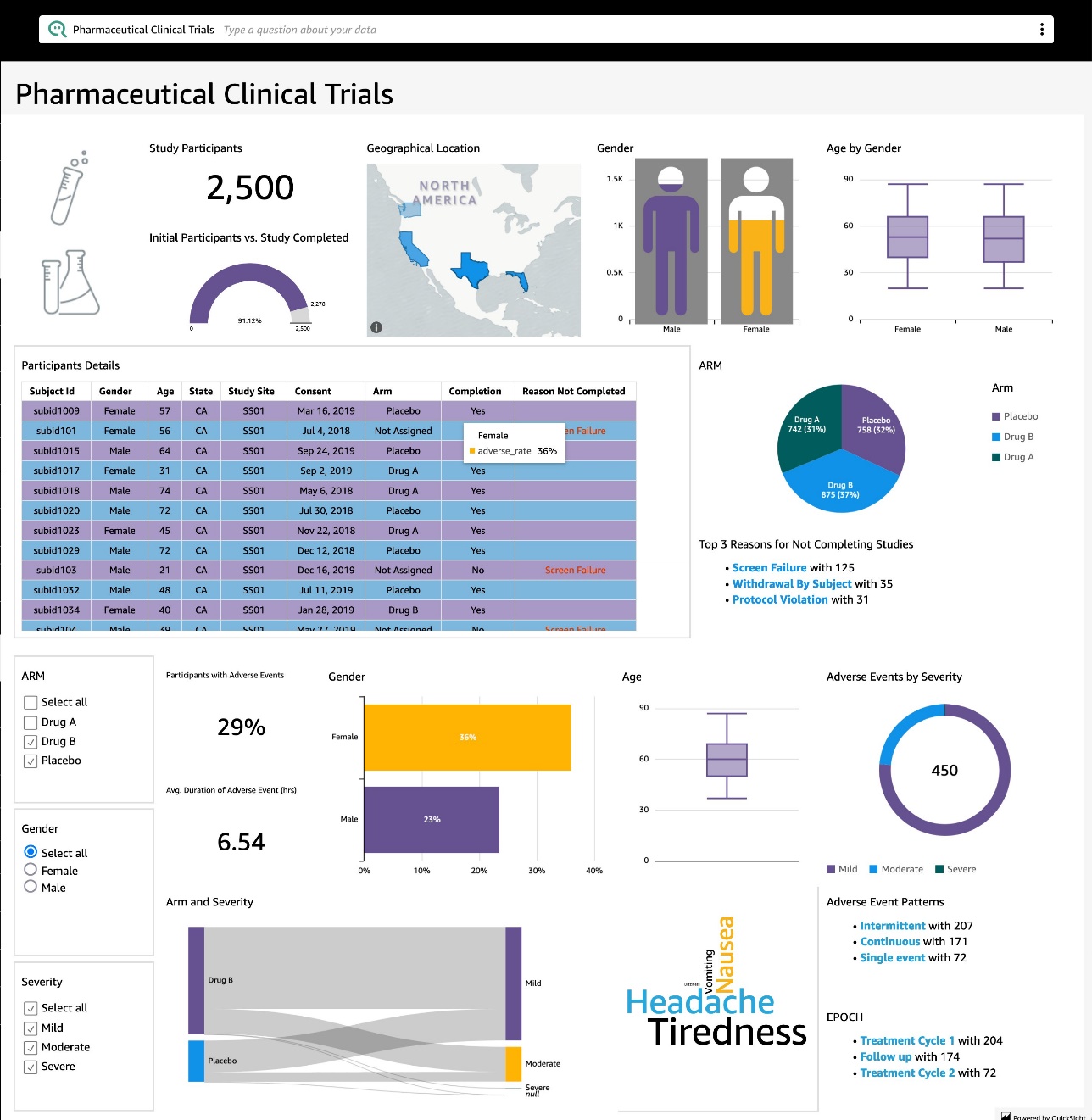
Allow customers to ask questions on knowledge utilizing pure language inside your functions by embedding Amazon QuickSight Q
Amazon QuickSight Q is a brand new machine learning-based functionality in Amazon QuickSight that permits customers to ask enterprise questions in pure language and obtain solutions with related visualizations immediately to realize insights from knowledge. QuickSight Q doesn’t rely upon prebuilt dashboards or stories to reply questions, which removes the necessity for enterprise intelligence (BI) groups to create or replace dashboards each time a brand new enterprise query arises. Customers can ask questions and obtain visible solutions in seconds instantly from inside QuickSight or from net functions and portals. On this publish, we take a look at how one can embed Q in your net functions or portals.
Resolution overview
Now you can embed Q in your utility with none customized improvement. Q is a completely managed cloud-native BI providing you could simply embed with out requiring experience inside your crew to develop and preserve this functionality. You possibly can simply democratize your knowledge and scale your insights to a rising person base, whereas guaranteeing you solely pay for utilization with Q’s distinctive pay-per-question pricing mannequin.
Purposes can authenticate customers with any id supplier of alternative (corresponding to Energetic Listing, Amazon Cognito, or any SAML-based federated SSO supplier that your group makes use of) and act on behalf of the person to get entry to the Q query bar. Which means that each person receives a safe, personalised query answering expertise whereas requiring no user-facing QuickSight-specific authentication. This permits a novel expertise to supply insights inside your utility with minimal upfront work and means that you can focus in your core utility performance! QuickSight Q embedding is on the market in QuickSight Enterprise Version and Q-supported Areas.
To facilitate a straightforward embedding expertise, AWS has additionally launched the Amazon QuickSight Embedding SDK (JavaScript) and a wealthy set of Q-specific functionalities. The QuickSight Embedding SDK permits you to effectively combine Q in your utility pages, set a default matter, allow matter choice, set themes, and management Q search bar conduct. This helps you roll out Q to your customers quicker.
To embed Q in your utility, you need to full the next high-level steps:
- Arrange permissions to generate embedded Q URLs.
- Generate a URL with the authentication code hooked up.
- Embed the Q search bar URL.
Arrange permissions to generate embedded Q URLs
On this step, you arrange permissions in your backend utility or net server to embed the Q search bar. This job requires administrative entry to AWS Identification and Entry Administration (IAM). Every person who accesses the Q search bar assumes a job that offers them QuickSight permissions to retrieve a Q-embedded URL.
To make this doable, create an IAM position in your AWS account. Affiliate an IAM coverage with the position to supply permissions to any person who assumes it. The IAM position wants to supply permissions to retrieve embedding URLs for a particular person pool. With the assistance of the wildcard character *
, you’ll be able to grant the permissions to generate a URL for all customers in a particular namespace. Or you’ll be able to grant permissions to generate a URL for a subset of customers in particular namespaces. For this, you add quicksight:GenerateEmbedUrlForRegisteredUser
.
The next pattern coverage offers these permissions:
Additionally, if you happen to’re creating first-time customers who can be QuickSight readers, make sure that so as to add the quicksight:RegisterUser
permission within the coverage.
The next pattern coverage offers permission to retrieve an embedding URL for first-time customers who can be QuickSight readers:
Lastly, your utility’s IAM id should have a belief coverage related to it to permit entry to the position that you simply simply created. Which means that when a person accesses your utility, your utility assumes the position on the person’s behalf and provisions the person in QuickSight.
The next instance makes use of a job referred to as embedding_quicksight_q_search_bar_role
, which has the pattern coverage previous as its useful resource:
Generate a URL with the authentication code hooked up
On this step, you authenticate your person and get the embeddable Q matter URL in your utility server. In the event you plan to embed the Q bar for IAM or QuickSight id sorts, share the Q matter with the customers. When a person accesses your app, the app assumes the IAM position of the person. If that person is new, the app provides the person to QuickSight, then passes an identifier because the distinctive position session ID.
These steps make it possible for every viewer of the Q matter is uniquely provisioned in QuickSight. It additionally enforces per-user settings, such because the row-level safety and dynamic defaults for parameters.
The next instance code performs the IAM authentication on the person’s behalf. This code runs in your app server:
To generate the URL you could embed in your app, name the GenerateEmbedUrlForRegisteredUser
API operation. This URL is legitimate for five minutes, and the ensuing session is legitimate for as much as 10 hours. You possibly can configure the session validity by setting the sessionLifetimeinMinutes
parameter for GenerateEmbedURL
APIs. The API operation offers the URL with an auth_code
worth that permits a single-sign on session. The next code exhibits an instance response from generate-embed-url-for-registered-user
:
Embed the Q search bar URL
On this step, you embed the Q search bar URL in your web site or utility web page. You are able to do this with the QuickSight Embedding SDK, which lets you do the next:
- Place the Q search bar on an HTML web page
- Move parameters into the Q search bar
- Deal with error states with messages which might be custom-made to your utility
Embed the Q search bar in your webpage by utilizing the QuickSight Embedding SDK or by including this URL into an iFrame. In the event you set a hard and fast top and width quantity (in pixels), QuickSight makes use of these and doesn’t change your visible as your window resizes. In the event you set a relative p.c top and width, QuickSight offers a responsive format that’s modified as your window measurement modifications. While you use the QuickSight Embedding SDK, the Q search bar in your web page is dynamically resized based mostly on the state. By utilizing the QuickSight Embedding SDK, you can even management parameters throughout the Q search bar and obtain callbacks when it comes to web page load completion and errors.
The next instance code exhibits how one can use the generated URL. This code is generated in your app server:
For this instance to work, make sure that to make use of the QuickSight Embedding SDK to load the embedded dashboard in your web site utilizing JavaScript. You may get the SDK within the following methods:
- Obtain the QuickSight Embedding SDK from GitHub. This repository is maintained by a bunch of QuickSight builders.
- Obtain the newest QuickSight Embedding SDK model from npmjs.com.
- In the event you use npm for JavaScript dependencies, obtain and set up it by operating the next command:
The next screenshot is an instance of the embedded Q query bar and a QuickSight dashboard to assist pure language questions and evaluation of pharmaceutical scientific trial knowledge. You possibly can check out this matter and different such matters in an embedded utility demo.
Abstract
Enterprises can empower customers to ask questions on knowledge in plain English inside their functions by embedding the QuickSight Q query bar. Embedding Q into your utility is simple and requires no customized improvement out of your crew.
Get began with a free trial of QuickSight Q.
Concerning the Authors
Deepak Murthy is a Senior Product Supervisor for Amazon QuickSight, AWS’s cloud-native, absolutely managed BI service. Deepak began his profession with Staples, creating enterprise knowledge warehouse options. Later, he was the architect of knowledge warehouse and analytics options at Wells Fargo, AMC, and Blackhawk Community. Deepak is happy concerning the potential of self-service analytics and bettering knowledge accessibility by enabling new pure language interactions with knowledge, and appears ahead to serving to prospects leverage these newest analytics improvements.
Rob Foley is a Software program Improvement Engineer for Amazon QuickSight, AWS’s cloud-native, absolutely managed BI service. Rob started his profession with AWS, and has been a member of the QuickSight crew for over 1.5 years. He has improvement expertise in a breadth of companies and stacks, primarily having labored on data-centric functions like Q.